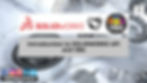
Introduction to SOLIDWORKS API and VBA
The SOLIDWORKS API (Application Programming Interface) allows you to automate and customize SOLIDWORKS using programming languages like VBA or C#. The API exposes the internals of SOLIDWORKS, enabling you to manipulate the user interface and automatically control things like parts, assembly, and drawing creation.
With the SOLIDWORKS API, you can significantly boost your productivity by eliminating repetitive manual steps in your design workflow. The API allows you to programmatically build components, generate drawings, set up assemblies, run simulations, and much more.
Some key benefits of using the SOLIDWORKS API for automation include:
- Reduced manual work and fewer errors
- Faster design iterations
- Ability to link SOLIDWORKS with other applications
- Create custom workflows specific to your industry
- Automatically generate documentation like BOMs
- Customize the SOLIDWORKS interface
- Enhanced productivity and efficiency
VBA (Visual Basic for Applications) is the programming language commonly used with the SOLIDWORKS API. VBA is built into SOLIDWORKS, making it easy to get started with API automation. With VBA, you can write macros that control SOLIDWORKS using simple commands like creating a new part or adding a feature.
VBA is a beginner-friendly language that doesn't require deep programming experience. The syntax is relatively easy to learn and many users are already familiar with VBA from Excel macros. So it's a great starting point for new API developers looking to automate their SOLIDWORKS workflow.
Installing the SOLIDWORKS API and VBA
The first step to start developing with the SOLIDWORKS API is to download and install the SOLIDWORKS SDK (Software Development Kit). This can be obtained on the SOLIDWORKS Customer Portal by logging in with your SOLIDWORKS credentials.
Once downloaded, run the SDK installation which will install the API help documentation, code samples, and necessary libraries. Make sure to select the version that matches your SOLIDWORKS release.
With the SDK set up, we next need to enable VBA macro settings in SOLIDWORKS. Go to Tools > Options > System Options > Macro Security and set the security level to "Enable Macros". This allows SOLIDWORKS to run the VBA code we will write.
The final requirement is to add a reference to the SOLIDWORKS Type Library which contains all the objects we can access in the API. In the VBA editor, go to Tools > References and check the box next to "SOLIDWORKS Type Library". Now VBA is connected to the SOLIDWORKS API.
We now have everything installed and configured to start programming with the SOLIDWORKS API using VBA macros! The SDK documentation and samples provide a wealth of information on how to use the extensive SOLIDWORKS automation capabilities. Let's start exploring ways to boost your productivity.
SOLIDWORKS API Fundamentals
The SOLIDWORKS API provides access to the SOLIDWORKS object model, allowing you to automate SOLIDWORKS using macros and programs. The key aspects of the SOLIDWORKS API include:
Object Model Overview
The SOLIDWORKS object model contains objects representing documents, parts, features, sketches, and other elements of SOLIDWORKS. The objects are organized in a hierarchical structure that you can traverse and interact with via code. For example, you can get a document object and, then from there access its parts, features, etc. Key objects include:
- SldWorks - The main SOLIDWORKS application object
- ModelDoc2 - Represents an open SOLIDWORKS document
- Feature - Base feature object type
- SketchSegment - Represents a sketch segment
By calling methods on these objects, you can automate SOLIDWORKS to perform tasks like creating features, adding sketches, generating drawings, and more.
Using the Macro Recorder
The macro recorder is a great way to start learning the SOLIDWORKS API. As you perform actions manually in SOLIDWORKS, the macro recorder logs the equivalent VBA code. You can then take that code and modify it to develop custom automation programs. The macro recorder lets you quickly see how to access objects like documents and translate manual interactions into API calls.
Help Documentation
The SOLIDWORKS API Help documentation provides detailed information on all available SOLIDWORKS objects and their members. It includes guides on getting started, tutorials, and reference material. The help documentation should be your primary reference as you learn how to use the SOLIDWORKS API. It contains code examples and explanations for every method and property available in the API.
Interacting with the SOLIDWORKS UI
The SOLIDWORKS API provides many options for customizing and interacting with the SOLIDWORKS user interface. This allows you to streamline your workflow by adding custom buttons, menus, and controls matched to your specific needs.
Customizing Toolbars and Menus
The SOLIDWORKS API includes objects for all the standard toolbars and menus. You can add and remove buttons, create flyout toolbars, and show/hide entire toolbars programmatically.
For example, to add a custom button to the CommandManager toolbar:
vb
Dim myButton As CommandManagerButton
Set myButton = CommandManagerView.ToolbarCommands.AddButton( _
"My Button", "MyMacro", "Runs My Macro", False)
This will insert a new button on the end of the toolbar that runs a macro called MyMacro. Icons and button styles can also be customized.
You can insert menu items in a similar way, by adding commands to the menu bar and context (right-click) menus. Flyout toolbars provide another option for organizing related buttons.
Adding Form Controls
Form controls like buttons, combo boxes, and text boxes can be embedded directly into the SOLIDWORKS GUI. These controls can run macros, show output data, or collect user input.
For example, to add a command button:
vb
Dim myButton As CommandButton
Set myButton = UserInterfaceManager.AddCommandButton("My Button", 500, 500, 100, 50)
myButton.SetMenuClickMacro "MyMacro"
Other form controls like editable text boxes, labels, combo boxes, and toggles can be added similarly. These controls open up many possibilities for interacting with users.
By leveraging the SOLIDWORKS API, you can customize the interface to maximize your productivity and streamline repetitive tasks. The sky's the limit for enhancing the user experience!
Selection and Navigation Techniques
Selecting objects in SOLIDWORKS models is a key aspect of API automation. The SOLIDWORKS API provides several tools and methods for selecting objects such as features, sketches, planes and more.
Selecting Objects in the FeatureManager Tree
The FeatureManager tree contains the history of the model. To select an object in the tree, first, get a reference to the FeatureManager object:
vb
Dim swApp As SldWorks.SldWorks = Application.SldWorks
Dim swFeatureManager As SldWorks.FeatureManager = Swapp.ActiveDoc.FeatureManager
Then use the FeatureManager object to access the required object. For example, to select a sketch:
vb
Dim swSketch As SldWorks.Sketch
swSketch = swFeatureManager.SketchManager.GetSketch2(1)
swSketch.Select2(True)
Similar methods exist for planes, features, assemblies and other objects.
Using Selection Filters
The API provides several filters that can narrow down a selection to specific types of objects. For example:
- swSelectByID - Select by unique ID
- swSelectByType - Select by object type
- swSelectByMaterial - Select by material
To use a filter:
vb
Dim selectionMgr As SldWorks.SelectionManager = swApp.ActiveDoc.SelectionManager
Dim selectionFilter As SldWorks.SelectData = selectionMgr.CreateSelectData
selectionFilter.AddFilter(swSelectByType, 0, 0, 0, 0)
selectionMgr.SelectByID2(selectionFilter, "Extrusion1", swAdd)
This selects the extrusion feature with ID "Extrusion1" using the swSelectByType filter.
Traversing the Model Tree
The SOLIDWORKS API includes methods for traversing the model tree and accessing child and parent objects. Key properties for traversal include:
- GetFirstChild2 - Gets first child object
- GetNextChild2 - Gets next child object
- GetParentFeature - Gets parent feature
For example:
vb
Dim swExtrusion = swFeatureManager.FeatureExtrusion3(1)
Dim swSketch = swExtrusion.GetFirstChild2(swSketchObject)
Dim swPlane = swSketch.GetParentFeature
This allows efficiently traversing the model hierarchy in API programs.
SOLIDWORKS Notifications
Notifications allow your VBA macros to be notified when certain events occur in SOLIDWORKS. This lets your macro respond to the event and take appropriate actions.
What are Notifications?
In SOLIDWORKS, notifications are a way for your macro to "listen" for specific events. When the event occurs, SOLIDWORKS will call your macro's notification handling function.
Some examples of notifications:
- Document open/close events
- Saving or activating a document
- Changes to dimensions or other parameters
- Starting a rebuild
- Selection changes
By subscribing to notifications, your macro can perform actions in response, such as updating models, capturing parameters, validating designs, etc.
Subscribing to Notifications
To subscribe to notifications in VBA:
1. Create a class module and define a function to handle the notification
2. Set the class module's Instancing property to "PublicNotCreatable"
3. Call IAFApplication.SubscribeToNotification to subscribe
For example:
vb
Public Class NotifyHandler
Public Sub OnDocumentOpen(ByVal Doc As Object)
MsgBox "Document opened!"
End Sub
End Class
Sub SubscribeToNotification()
Dim handler As New NotifyHandler
IAFApplication.SubscribeToNotification NotifyDocumentOpened, handler
End Sub
When the NotifyDocumentOpened event occurs, SOLIDWORKS will call the OnDocumentOpen handler function.
Common Notification Use Cases
Here are some common ways SOLIDWORKS notifications are used in automation:
- Updating custom properties when the model changes
- Capturing dimension values on rebuild
- Validating models when documents are saved
- Responding to selection changes
- Performing actions when new components are inserted
By leveraging notifications, you can create macros that dynamically respond to changes in SOLIDWORKS, instead of just scripted actions. This makes automation more robust and adaptable.
Notifications allow the creation of complex interactions between your macros and the SOLIDWORKS API. They are a key capability for automation.
Automating Parts in SOLIDWORKS
SOLIDWORKS provides many methods and objects to automate the creation of parts programmatically. Here we will explore techniques for automating sketches, features, relations and dimensions when developing parts with VBA and the SOLIDWORKS API.
Creating Sketches and Features
The first step in automating part design is creating sketches. The SketchManager object provides methods like Add and CreateCornerRectangle to construct the sketch geometry. For example:
vb
Dim sm As SketchManager = Part.SketchManager
Dim skch As Sketch = sm.Add(Part.ActiveBody)
Dim rect As CornerRectangle = skch.CreateCornerRectangle(0, 0, 100, 75)
Once you have a sketch, you can add features like extrusions. The FeatureManager object manages adding features:
vb
Dim fm As FeatureManager = Part.FeatureManager
Dim extrude As ExtrudedBossFeature = fm.ExtrudedBossFeature(rect)
extrude.EndOffsetDistance = 50
extrude.BossEndCondition = EndConditions.Blind
Similar methods exist for creating other features like fillets, holes, drafts, etc.
Adding Relations and Dimensions
To properly constrain sketches, you need relations and dimensions. The SketchManager provides methods for this:
vb
Dim con As SketchConstraint = skch.AddConstraint(rect, rect.GetEdges(), _
SketchConstraintType.horizontal)
Dim As SketchDimension = such.AddDimension(rect, rect.GetEdges(), 0)
Set dimension values and add other relations like coincident, parallel, etc. The API provides full control over sketch constraints.
Best Practices
When automating parts, follow these best practices:
- Fully constrain sketches to avoid failed features
- Use depth-first rather than breadth-first order for features
- Check errors after adding each feature
- Keep feature names and references for rebuilding
- Use robust naming conventions for consistency
Following these practices will create reliable, reusable automated parts.
Automating Assemblies in SOLIDWORKS
The SOLIDWORKS API provides many ways to automate the creation and management of assemblies. This enables you to programmatically build assemblies in an automated fashion for improved productivity.
Some key capabilities for assembly automation include:
Inserting Components and Mates
You can insert existing SOLIDWORKS parts and sub-assemblies into an assembly using VBA. The key steps are:
1. Call Swapp.ActiveDoc to get the active assembly document.
2. Use AddComponent3 to select and insert a part or sub-assembly.
3. Add mates between components using Mate2, MateAlign, etc.
4. Set mate references with functions like Set2dSketchAxis.
This allows you to quickly insert components and add mates through code.
Managing Large Assemblies
The SOLIDWORKS API includes many tools to help work with large assemblies more efficiently:
- Use IAssemblyDoc::GetComponents to get all components. You can then loop through them and process them as needed.
- Lightweight mode streamlines opening and processing assemblies.
- Skip load improves rebuild time by only loading visible components.
- The IModelDocExtension interface aids in managing external references.
Top-Down vs Bottom-Up Design
You can take both top-down and bottom-up approaches:
- Top-down: Create assembly structure first, then add parts to fill it in.
- Bottom-up: Create parts first, then combine them into the assembly.
The API gives flexibility to automate either way.
Overall, the SOLIDWORKS API provides many features to automate assembling components, streamline working with large assemblies, and support different design approaches.
Automating Drawings in SOLIDWORKS
The SOLIDWORKS API provides many ways to automate the creation and customization of drawings. This enables you to efficiently generate production-ready drawings from your CAD models.
Creating Drawings from Models
You can use the API to programmatically create new drawings from existing parts and assemblies. The key steps are:
- Call ISldWorks::NewDrawing to create a new drawing document.
- Open the model you want to base the drawing on.
- Use IModelDoc2::GetViewByName to retrieve a default model view.
- Add the view to the drawing with IDrawingDoc::InsertView.
This will insert a base view into the drawing. You can then configure the scale, display states, and other properties of the view.
Adding Views, Annotations, and Tables
Beyond the base model view, you can insert additional projected, section, and detail views. The API includes methods like IDrawingDoc::InsertProjectedView and IDrawingDoc::InsertSectionView to automate the addition of these drawing views.
To annotate the drawing, you can add dimensions, notes, hole callouts, and other annotations. The API provides specialized methods for adding each type, like IDrawingDoc::AddDimension3, IDrawingDoc::AddNote, and IDrawingDoc::AddHoleCallout.
For tables, you can insert parts lists, hole tables, weldment cut lists, and more. Use IDrawingDoc::InsertTable and specify the type of table.
Customizing Templates
The drawing template in SOLIDWORKS controls the formatting and style of new drawings. You can customize templates programmatically by adding custom properties, and logos, and changing document settings.
Modify template properties using IDrawingDoc::SetCustomProperty. To add a logo, insert it as a drawing view with IDrawingDoc::InsertView. For document settings, use IDrawingDoc::SetSetting.
This allows you to automate the creation of drawings that match your company or industry standards.
Adding Custom Properties
One powerful feature of the SOLIDWORKS API is the ability to create custom properties that can store custom data on parts, assemblies, and drawings. Custom properties allow you to add additional metadata that is not included in the standard SOLIDWORKS properties.
Defining Custom Properties
To define a new custom property, you use the IModelDocExtension interface's AddCustomProperty2 method. This allows you to specify a name, type, value, and other attributes for the new property. Some examples of types for custom properties are integer, float, bool, string, date, and color.
Here is an example of using VBA to add a custom integer property called "RevisionNumber":
vb
Dim swDoc As SldWorks.ModelDoc2
Dim customProp As Property
Set swDoc = ThisApplication.ActiveDoc
Set customProp = swDoc.Extension.AddCustomProperty2( _
"RevisionNumber", swCustomInfoType_e.swCustomInfoType_Int32)
customProp.ValInt32 = 1
Setting and Retrieving Property Values
Once a custom property is defined, you can use the property's value setting and retrieval methods to assign and read values from it. The methods available depend on the type of the property. For example, with an integer property like the one above, you would use ValInt32 to set and get the value.
To retrieve all custom properties, you can use the GetAllCustomProperties method. This returns a collection of Property objects representing each defined custom property.
Using Attributes to Populate Properties
Attributes provide an automated way to populate custom properties and other fields when a new document is created from a template. You add attributes to the template document, then the attribute values get filled in with data when a new document is made.
For example, you could create a "PartNumber" attribute in a part template. New parts made from that template would automatically have the "PartNumber" custom property populated with a unique part number value.
Attributes help streamline workflows where certain metadata fields need to be consistently defined on new documents. The API provides full control over creating, editing, and reading attributes programmatically.